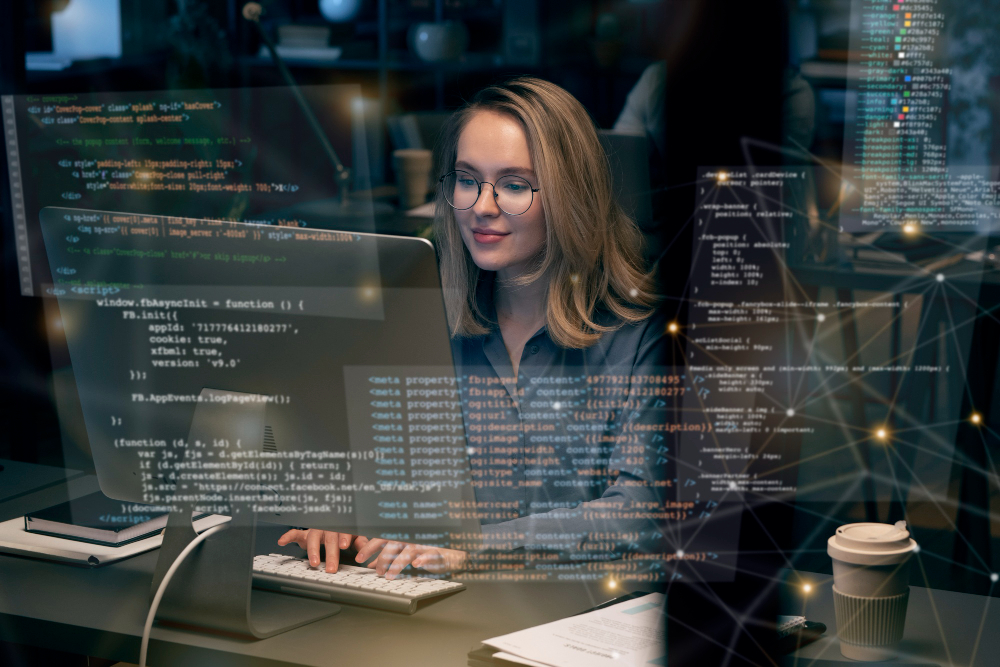
comprehensive syllabus for a Full Stack Development course, starting from the basics to advanced topics. The curriculum covers both front-end and back-end technologies, databases, deployment, and more.
- Introduction to Full Stack Development
- What is Full Stack Development?
- Front-end vs Back-end vs Full Stack
- Tools and Software Setup
- IDEs (e.g., VS Code)
- Version Control (Git, GitHub)
- Front-end Development
HTML (Hypertext Markup Language)
- Structure of an HTML Document
- Semantic Elements
- Forms and Input Elements
- Media (Images, Audio, Video)
- Accessibility Best Practices
CSS (Cascading Style Sheets)
- Selectors and Specificity
- Box Model
- Flexbox and Grid Layouts
- Media Queries (Responsive Design)
- CSS Frameworks (Bootstrap, Tailwind CSS)
JavaScript (Client-Side Scripting)
- Variables and Data Types
- Loops, Conditionals, and Functions
- DOM Manipulation
- Events and Event Listeners
- ES6+ Features (Arrow Functions, Promises, Modules)
- Fetch API and AJAX
Front-end Frameworks and Libraries
- Introduction to React.js
- Components and Props
- State and Lifecycle
- React Hooks
- Routing with React Router
- Introduction to Angular or Vue.js (optional)
- Version Control and Collaboration
- Git Basics (Commit, Push, Pull, Branching)
- Collaborating with GitHub
- Resolving Merge Conflicts
- Back-end Development
Programming Languages
- Introduction to Node.js
- Setting up a Server
- Working with Modules
- Building RESTful APIs
- Introduction to Python with Flask/Django (optional)
Databases
- SQL Basics
- CRUD Operations
- Joins and Subqueries
- Database Design and Normalization
- NoSQL Databases
- MongoDB Basics
- Collections and Documents
- Aggregation Framework
Authentication and Authorization
- Password Hashing
- JWT (JSON Web Tokens)
- OAuth 2.0
- API Development
- RESTful APIs
- CRUD Operations
- Status Codes
- Middleware
- Graph QL Basics (optional)
- DevOps and Deployment
- Introduction to Web Servers
- Apache/Nginx Basics
- Hosting Platforms
- Heroku, Netlify, or Vercel
- AWS Basics
- Continuous Integration/Continuous Deployment (CI/CD)
- Advanced Topics
- State Management (Redux or Context API in React)
- WebSocket’s (Real-Time Communication)
- Progressive Web Apps (PWAs)
- Testing and Debugging
- Unit Testing with Jest
- Debugging Tools in Browsers
- Performance Optimization
- Lazy Loading
- Code Splitting
- Caching Strategies
- Capstone Project
- Building a Full-Stack Application
- Example: E-commerce Website, Blog Platform, Social Media App
- Group Collaboration with GitHub
- Final Deployment and Presentation
- Soft Skills for Developers
- Writing Technical Documentation
- Code Reviews and Best Practices
- Communication in Agile Teams
This syllabus provides a clear path for aspiring full-stack developers, ensuring a solid foundation in web development and real-world experience through projects and collaboration.